The introduction of Gutenberg (Block Editor) to WordPress was a revolutionary change for the most popular CMS. Thus, creating custom blocks is one of the most exciting tasks for the modern WordPress Developer.
Gutenberg is built on React. So it is highly recommended to use React in order to create custom blocks.
ClioWP Blocks Boilerplate (as the name suggests) is a “starter” block-type plugin that generates a message that the user can customize:
- the message itself (obviously) – by default “Hello Gutenberg!”
- the border-color
- the background color
- the message alignment
It can be the basis for creating your own Gutenberg Plugin.
In Greek mythology, Clio is the muse of history: https://en.wikipedia.org/wiki/Clio
Download
Download the latest release from https://github.com/pontikis/cliowp-blocks-boilerplate/releases
Characteristics
- It is based on React (functional components) and ES6 techniques
- It is registered based on block.json
- Teaches you how to manipulate parameters from the Settings Sidebar of Gutenberg (
InspectorControls
) - Teaches you how to manage the Popup Toolbar in Gutenberg (
BlockControls
) - It is built based on Industry Standard Development Tools
- It follows the WordPress Coding Standards
- It has multilingual support (i18n)
- It is Free, Open-Source, and available under the GPLv2
Screenshots
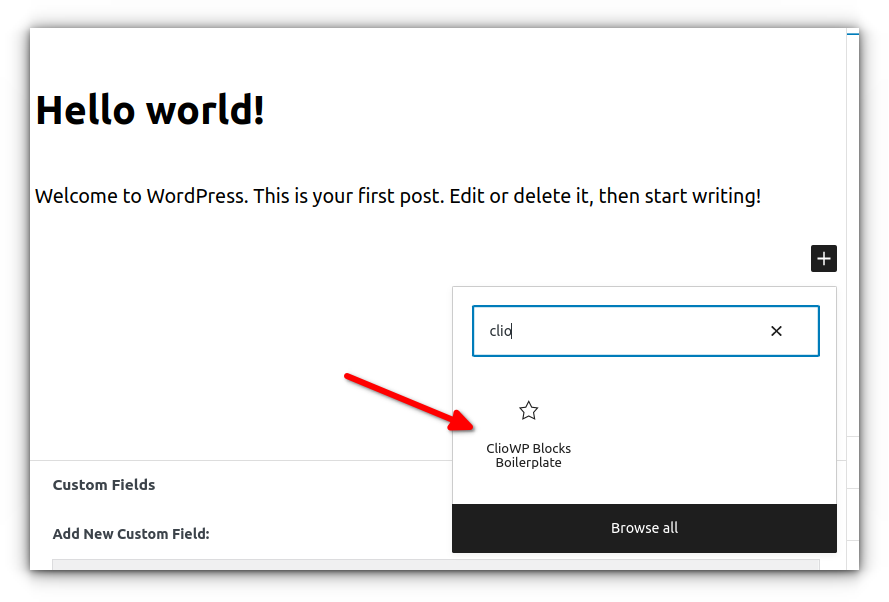
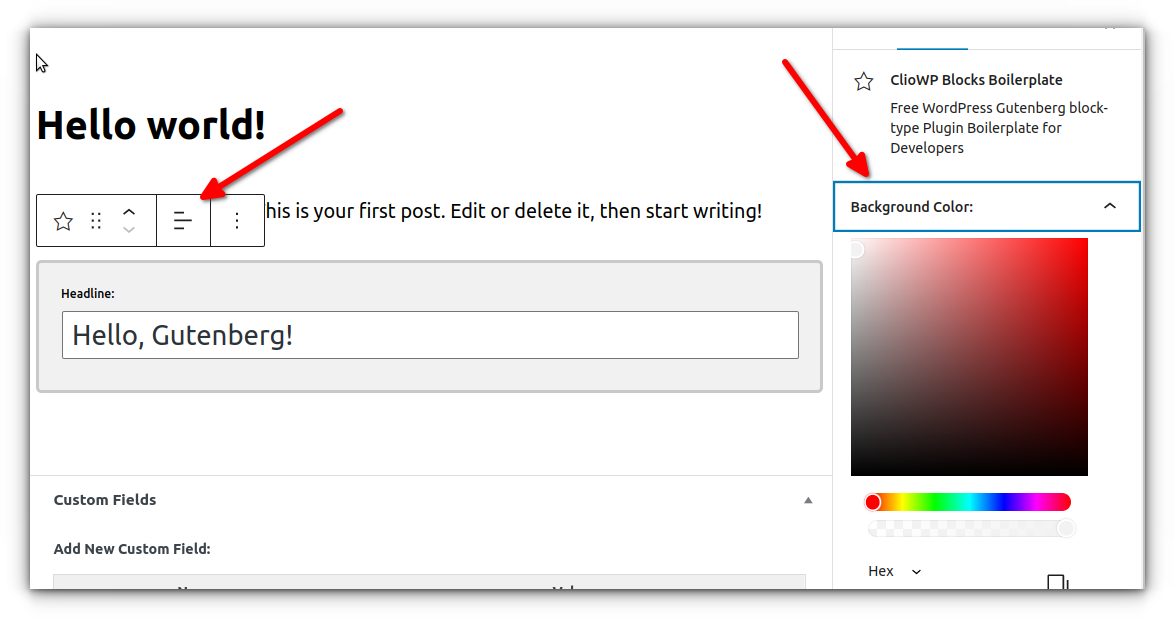
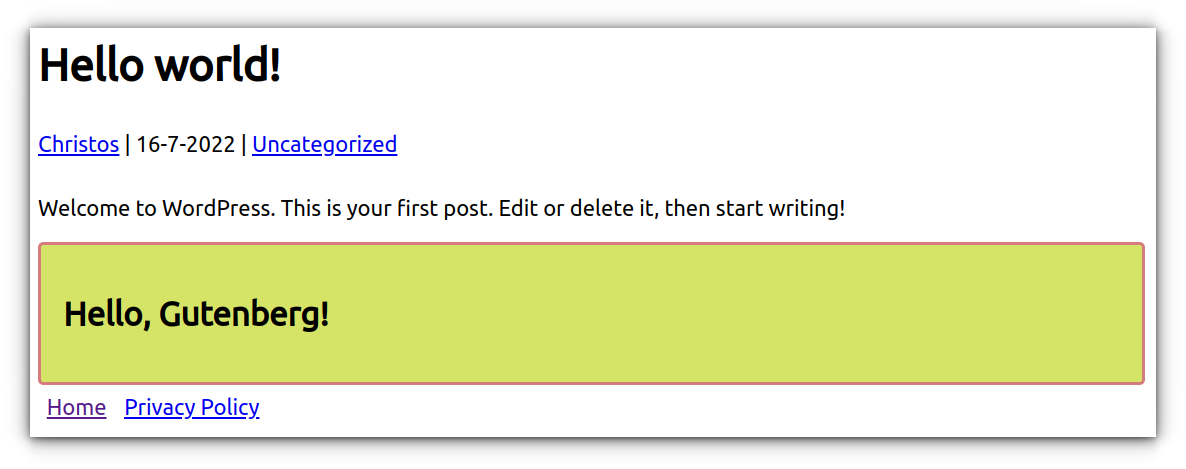
Plugin structure
(node_modules
and language files are omitted)
.
├── block.json
├── build
│ ├── editor.asset.php
│ ├── editor.css
│ ├── editor.css.map
│ ├── editor.js
│ ├── editor.js.map
│ ├── frontend.asset.php
│ ├── frontend.css
│ ├── frontend.css.map
│ ├── frontend.js
│ └── frontend.js.map
├── class-cliowp-blocks-boilerplate.php
├── css
│ ├── editor.scss
│ ├── frontend.scss
│ └── modules
│ ├── editor
│ │ └── main.scss
│ └── frontend
│ └── main.scss
├── languages
├── LICENSE
├── package.json
├── package-lock.json
├── README.md
├── readme.txt
└── src
├── editor.js
├── frontend.js
└── modules
├── editor
│ ├── EditBlock.js
│ └── RegisterBlock.js
└── frontend
├── DisplayBlock.js
└── MyComponent.js
The main PHP file class-cliowp-blocks-boilerplate.php
As in any WordPress plugin, we need the main PHP file, where:
- the plugin metadata are defined in the top comments
- a PHP class is defined and an instance of this class is created
- we tell to WordPress to load our Javascript code (in Gutenberg and probably in the front end). We use the register_block_type() function (or the register_block_type_from_metadata()).
- we define a PHP callback function (optional, but recommended). PHP callback will create the result in the front end dynamically, except you only need static content.
The block.json
file
block.json
(in the root of your plugin folder) is the canonical way to register a block-type plugin since WordPress 5.8
This file allows your plugin to be included in the WordPress block directory https://wordpress.org/plugins/browse/block/
Also, it makes JS and CSS assets management easier.
{ "$schema": "https://schemas.wp.org/trunk/block.json", "apiVersion": 2, "name": "cliowp-blocks/boilerplate", "title": "ClioWP Blocks Boilerplate", "category": "common", "icon": "star-empty", "description": "Free WordPress Gutenberg block-type Plugin Boilerplate for Developers", "attributes": { "headline": { "type": "string", "default": "Hello, Gutenberg!" }, "bgColor": { "type": "string", "default": "#f1f1f1" }, "borderColor": { "type": "string", "default": "#cac8c8" }, "headlineAlignment": { "type": "string", "default": "left" } }, "example": { "headline": "Hello, Gutenberg!", "bgColor": "#f1f1f1", "borderColor": "#cac8c8", "headlineAlignment": "left" }, "textdomain": "td-cliowp-blocks-boilerplate", "editorScript": "file:./build/editor.js", "editorStyle": "file:./build/editor.css", "script": "file:./build/frontend.js", "style": "file:./build/frontend.css" }
editorScript
: Javascript will only be enqueued in the context of the editor.editorStyle
: CSS will only be enqueued in the context of the editor.script
: Javascript will be enqueued both in the editor and the front end.style
: CSS will be enqueued both in the editor and the front end.icon
: from Dashicons without the dashicon- prefix https://developer.wordpress.org/resource/dashicons
As you can see in the official documentation
- there is a
viewScript
property, but it will not be used if you are using a PHP render callback - there is not (at least for now) a
viewStyle
property (WordPress/gutenberg#41236)
So, I use script
and style
for front-end assets.
Reference: https://developer.wordpress.org/block-editor/reference-guides/block-api/block-metadata/
The back-end javascript (editor.js
)
We use registerBlockType from @wordpress/blocks to register the block.
- the edit function is responsible for the structure of the block in the context of the Gutenberg Editor
- the save function is responsible for the front-end result. In my case, it returns
null
, as the PHP callback is responsible to create the front-end dynamically
The front-end javascript (frontend.js
)
I use a React component in the front end. So, you can create impressive and interactive blocks, except you only need static content.
i18n
- i18n PHP – load_plugin_textdomain() and esc_html__()
- i18n JS – generate_block_asset_handle() and wp_set_script_translations().
I use Loco translate for translations.
How to use it
In order to create a custom Gutenberg block, actually, you have to create a plugin. A block-type WordPress plugin (here is the official documentation).
- Create a folder in wp-content/plugins for your block-type plugin
- Copy boilerplate in this folder
- Customize metadata in the comments section of the main PHP file
- Customize metadata in the
block.json
- Define your project with
npm init
(this will createpackage.json
file) - Install @wordpress/scripts
npm install @wordpress/scripts --save-dev
- Add
start
andbuild
scripts in package.json
"scripts": { "start": "wp-scripts start src/editor.js src/frontend.js", "build": "wp-scripts build src/editor.js src/frontend.js", "test": "echo "Error: no test specified" && exit 1" },
- Run
npm run start
Details at: https://www.pontikis.net/blog/how-to-write-js-and-css-in-wordpress-with-industry-standard-tools
References
- https://developer.wordpress.org/block-editor/getting-started/create-block/
- https://developer.wordpress.org/block-editor/how-to-guides/block-tutorial/writing-your-first-block-type/
- https://developer.wordpress.org/block-editor/how-to-guides/block-tutorial/block-controls-toolbar-and-sidebar/
Internationalization – i18n
- How to use i18n in JavaScript
- New! JavaScript i18n support in WordPress 5.0
- https://wordpress.stackexchange.com/questions/407346/using-wp-set-script-translations-without-manually-registering-the-script
- Registering block with block.json not load translation #34478
- wp.i18n.esc_html__() version? XSS? #12220