Debugging is an essential part of Flutter development, helping developers uncover and resolve issues in their applications. In this guide, we’ll explore various debugging techniques, tools, and best practices specifically tailored for Flutter developers. From effective logging to powerful debugging tools like Dart Observatory and Flutter Inspector, we’ll cover basic and advanced techniques for efficient bug identification and resolution. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge and tools to become a proficient debugger in Flutter. Let’s uncover the secrets of effective debugging together.
Here is a blog on Debugging Tips and Tricks for Flutter Developers
Using print statements
Print statements are a simple way to debug Flutter apps. They can be used to print out the value of a variable or expression, which can be helpful for debugging. For example, if you are trying to figure out why a variable is not being set correctly, you can use a print statement to print out the value of the variable.
To use a print statement, you simply need to add the print() function to your code. The print() function takes a string as input, and it will print that string to the console. For example, the following code will print the value of the variable myVar to the console:
Code snippet
int myVar = 10;
print(myVar);
Using breakpoints
Breakpoints are another useful debugging tool. Breakpoints allow you to stop the execution of your code at a specific point. This can be helpful for debugging because you can then inspect the values of variables and expressions at that point in the code.
To set a breakpoint, you need to click on the left margin of the code editor, at the line where you want to set the breakpoint. A red dot will appear at the line, indicating that a breakpoint has been set.
When your code reaches the breakpoint, the execution of the code will stop. You can then inspect the values of variables and expressions at that point in the code.
The Flutter Inspector
The Flutter Inspector is a visual tool that allows you to inspect the state of your Flutter app. You can use the Inspector to view the widget tree, the rendering tree, and the state of individual widgets.
The Inspector is divided into two main sections: the Widget Tree and the Rendering Tree.
The Widget Tree shows the hierarchy of widgets that make up your app. You can use the Widget Tree to find specific widgets, see how they are related to each other, and inspect their properties.
The Rendering Tree shows how the widgets in your app are rendered on the screen. You can use the Rendering Tree to see how the widgets are positioned, to see how they are being sized, and to see how they are being decorated.
In addition to the Widget Tree and the Rendering Tree, the Inspector also includes a number of other tools that can help you debug your app. These tools include:
- The Properties Inspector, allows you to inspect the properties of individual widgets.
- The Logs, which shows the output of the Flutter logging system.
- The Breakpoints, which allow you to stop the execution of your code at a specific point.
The DevTools
The DevTools are a set of debugging tools that are available for Flutter apps. The DevTools include a debugger, a profiler, and a performance inspector.
The Debugger allows you to step through your code line by line, inspect the values of variables, and set breakpoints.
The Profiler allows you to measure the performance of your app. You can use the Profiler to see how much time is being spent in different parts of your code and to identify bottlenecks.
The Performance Inspector allows you to see how your app is performing on the device. You can use the Performance Inspector to see how much memory is being used, how much CPU is being used, and how much network traffic is being generated.
The DevTools are a powerful set of tools that can help you debug your Flutter apps. If you are having trouble debugging your app, I recommend that you try using DevTools.
Conclusion
Mastering debugging techniques is crucial for Flutter developers to identify and resolve issues in their applications efficiently. By effectively utilizing logging, leveraging powerful debugging tools like Dart Observatory and Flutter Inspector, and applying advanced techniques such as network analysis and breakpoints, developers can streamline the debugging process and enhance their Flutter development skills. Whether you’re a beginner or an experienced developer, the insights and best practices shared in this guide will enable you to overcome challenges and deliver high-quality Flutter development services.
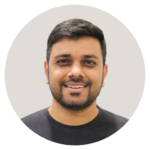
Pramesh Jain, CEO, TechnoPreneur, at WebMob Technologies, has an experience of over 12 years. He is the intellectual head of software solutions with expertise in client acquisition, project inception, & strategic application growth development. Embracing every software trend and developing seamless applications is his passion.