http is a stateless protocol. A way to remember information from page to page is to use cookies. According to Wikipedia:
A cookie, also known as an HTTP cookie, web cookie, or browser cookie, is a small piece of data sent from a website and stored in a user’s web browser while the user is browsing that website. Every time the user loads the website, the browser sends the cookie back to the server to notify the website of the user’s previous activity. Cookies were designed to be a reliable mechanism for websites to remember stateful information (such as items in a shopping cart) or to record the user’s browsing activity (including clicking particular buttons, logging in, or recording which pages were visited by the user as far back as months or years ago).
In this tutorial you will find information about creating and managing cookies with PHP or Javascript.
Create cookies with PHP
(REMARK: for a visual way to display the following actions, see below “Manage cookies in various web browsers”).
Create
Use setcookie to create a cookie with PHP. This cookie will expire after 30 days. Using “/”, cookie is available in all website (otherwise, select the directory you prefer).
$cookie_name = 'pontikis_net_php_cookie';
$cookie_value = 'test_cookie_set_with_php';
setcookie($cookie_name, $cookie_value, time() + (86400 * 30), '/'); // 86400 = 1 day
Read
Use $_COOKIE to retrieve a cookie with PHP. Once the cookies have been set, they can be accessed on the next page load.
$cookie_name = 'pontikis_net_php_cookie';
if(!isset($_COOKIE[$cookie_name])) {
print 'Cookie with name "' . $cookie_name . '" does not exist...';
} else {
print 'Cookie with name "' . $cookie_name . '" value is: ' . $_COOKIE[$cookie_name];
}
Update
Actually, there is not a way to update a cookie. Just set (again) this cookie using setcookie.
$cookie_name = 'pontikis_net_php_cookie';
$cookie_value = 'test_cookie_updated_with_php';
setcookie($cookie_name, $cookie_value, time() + (86400 * 30), '/'); // 86400 = 1 day
Delete
Actually, there is not a way to directly delete a cookie. Just use setcookie with expiration date in the past, to trigger the removal mechanism in your browser.
$cookie_name = 'pontikis_net_php_cookie';
unset($_COOKIE[$cookie_name]);
// empty value and expiration one hour before
$res = setcookie($cookie_name, '', time() - 3600);
Create cookies with Javascript
(REMARK: for a visual way to display the following actions, see below “Manage cookies in various web browsers”).
Create
Using document.cookie with following format:
document.cookie="name_here=value_here; expires=date_in_UTC_here; path=path_here";
You many use a function like this one:
/**
* Create cookie with javascript
*
* @param {string} name cookie name
* @param {string} value cookie value
* @param {int} days2expire
* @param {string} path
*/
function create_cookie(name, value, days2expire, path) {
var date = new Date();
date.setTime(date.getTime() + (days2expire * 24 * 60 * 60 * 1000));
var expires = date.toUTCString();
document.cookie = name + '=' + value + ';' +
'expires=' + expires + ';' +
'path=' + path + ';';
}
So, to create a cookie which will expire after 30 days:
var cookie_name = 'pontikis_net_js_cookie';
var cookie_value = 'test_cookie_created_with_javascript';
create_cookie(cookie_name, cookie_value, 30, "/");
Read
document.cookie will return all cookies in one string as following: cookie1=value; cookie2=value; cookie3=value;. So to get the value of a cookie you have to parse this string.
You many use a function like this one:
/**
* Retrieve cookie with javascript
*
* @param {string} name cookie name
*/
function retrieve_cookie(name) {
var cookie_value = "",
current_cookie = "",
name_expr = name + "=",
all_cookies = document.cookie.split(';'),
n = all_cookies.length;
for(var i = 0; i < n; i++) {
current_cookie = all_cookies[i].trim();
if(current_cookie.indexOf(name_expr) == 0) {
cookie_value = current_cookie.substring(name_expr.length, current_cookie.length);
break;
}
}
return cookie_value;
}
So, to retrieve a cookie with name “pontikis_net_js_cookie”:
var cookie_name = 'pontikis_net_js_cookie';
var res = retrieve_cookie(cookie_name);
if(res) {
alert('Cookie with name "' + cookie_name + '" value is ' + '"' res + '"');
} else {
alert('Cookie with name "' + cookie_name + '" does not exist...');
}
Update
Just, recreate cookie with new values:
var cookie_name = 'pontikis_net_js_cookie';
var cookie_name = 'test_cookie_updated_with_javascript';
create_cookie(cookie_name, cookie_value, 60, "/");
Delete
Just give an expiration date in the past. Cookie value not needed.
document.cookie = "pontikis_net_js_cookie=; expires=Thu, 01 Jan 1970 00:00:00 GMT; path=/";
You many use a function like this one:
/**
* Delete cookie with javascript
*
* @param {string} name cookie name
*/
function delete_cookie(name) {
document.cookie = name + "=; expires=Thu, 01 Jan 1970 00:00:00 GMT; path=/";
}
So, to delete a cookie:
var cookie_name = 'pontikis_net_js_cookie';
delete_cookie(cookie_name);
Create cookies with jQuery
There is a jQuery plugin available here https://github.com/carhartl/jquery-cookie.
Manage cookies in various web browsers
Google Chrome
Go to “Chrome Developer Tools” (Menu → Tools → Developer Tools or press CTRL+SHIFT+I). Go to “Resources” tab and open “Cookies” section
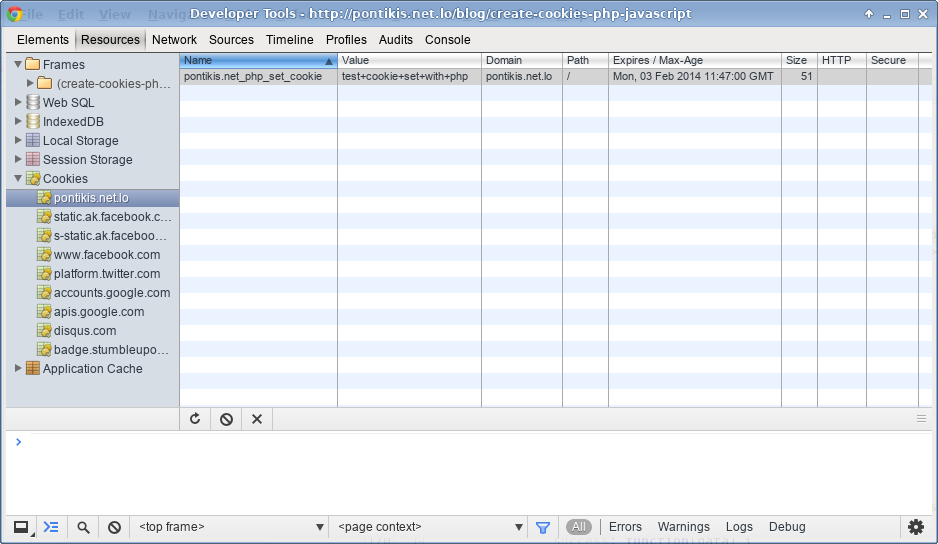
Mozilla Firefox
Go to “Firefox Preferences” (Menu → Tools → Options on Windows or Menu → Edit → Preferences on Linux). Go to “Privacy” tab and press “Show Cookies”
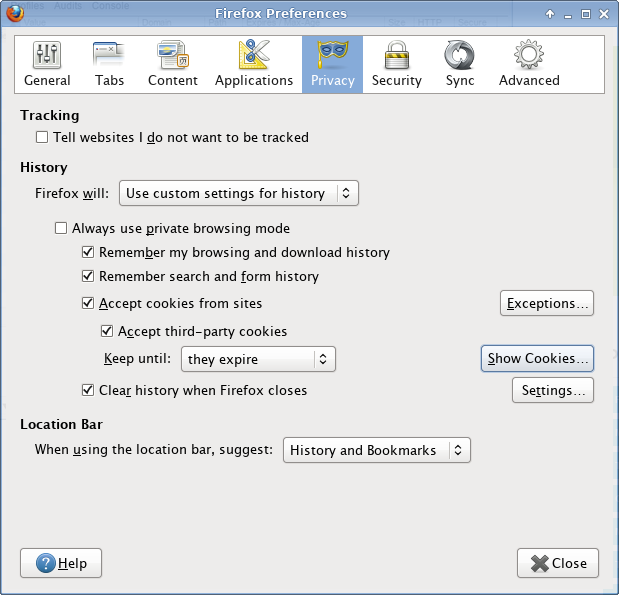
here is the cookie
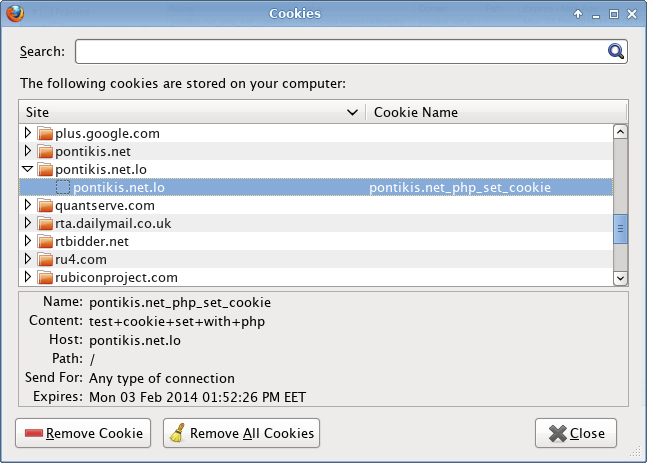
Another way is to install a Firefox plugin. A nice plugin is “View Cookies”. Get it here:
Go to “Page Info” (Menu → Tools → Page info) or just press “CTRL+I”. A new tab (“Cookies”) is present:
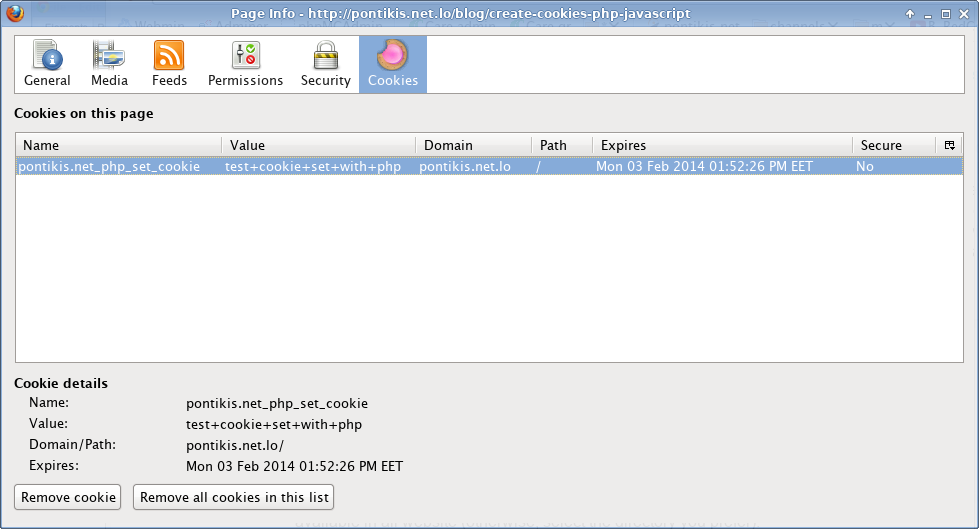
Internet Explorer
It is less convenient to view cookies in Microsoft Internet Explorer
Go to “Internet Options” (Menu → Internet Options). Go to first tab “General”. Click “Settings” and then “View files”
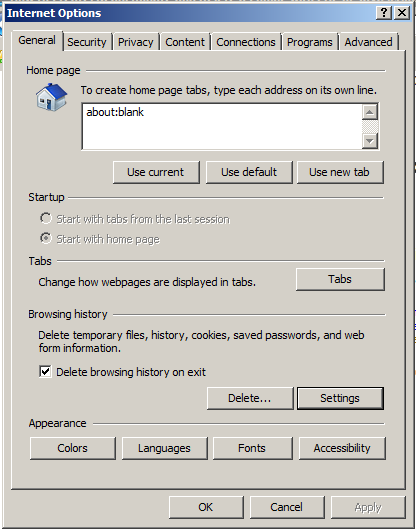
Scroll down this list until you see the files labeled as cookies
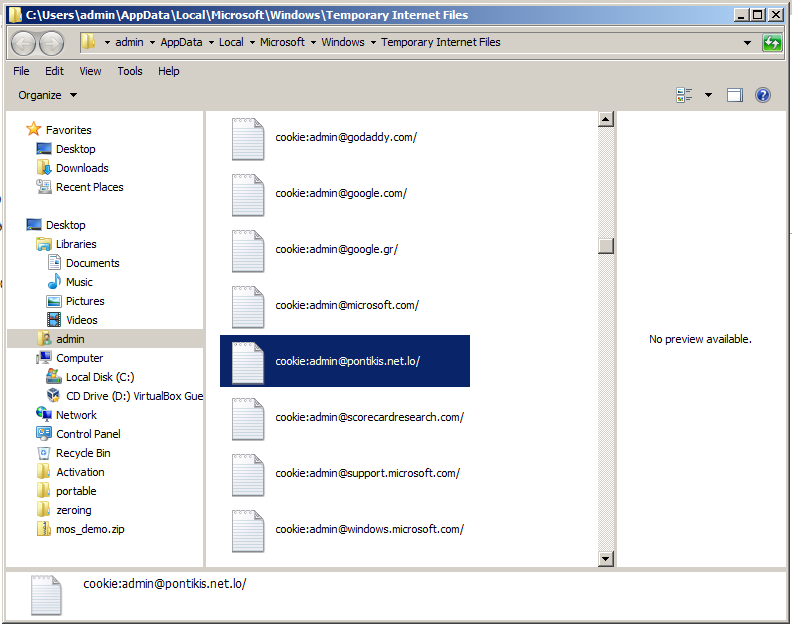
There is also an utility available (IECookiesView) More details here.
Enable or disable cookies
Every user can disable browser cookies. But, keep in mind that most websites will not work properly without cookies.
Instructions for major browsers are available here:
- Enable or disable cookies in Google Chrome
- Enable or disable cookies in Mozilla Firefox
- Enable or disable cookies in Internet Explorer
Check if cookies are enabled
Check if cookies are enabled with PHP
Try to create a test cookie using setcookie and then count $_COOKIE array:
setcookie('check_cookies_enabled', 'test', time() + 3600, '/');
if(count($_COOKIE) > 0) {
print 'Cookies are enabled!';
} else {
print 'Cookies are disabled...';
}
Check if cookies are enabled with Javascript
You can rely to navigator.cookieEnabled, as it supported from all major browsers:
if(navigator.cookieEnabled) {
alert('Cookies are enabled!');
} else {
alert('Cookies are disabled...');
}
Entrepreneur | Full-stack developer | Founder of MediSign Ltd. I have over 15 years of professional experience designing and developing web applications. I am also very experienced in managing (web) projects.